こんにちはーー
YouTubeの英語動画を文字起こしして自動翻訳するプログラムを作成しました。
この記事が誰かの役に立てば幸いです。
動画にもしていますーー
対象の動画
適当に↓のアメリカ株に関連する動画に対して実行します。
プログラムの概要
ファイル名 | 実行している内容 |
main.py | 共通の変数定義、作成した関数の実行 |
dw_mp3.py | Youtubeから音声ファイル(mp3)をダウンロード |
conv.py | ダウンロードしたmp3をwavに変換 |
c_wav.py | 容量の重いファイルは文字起こしできないためwavを分割 |
w_csv.py | wavそれぞれに文字起こしを実行 |
conv_xlsx.py | 編集しやすいようにcsvからエクセルファイルに変換 |
trans_jpn.py | 英語を翻訳 |
作成したスクリプトの一覧
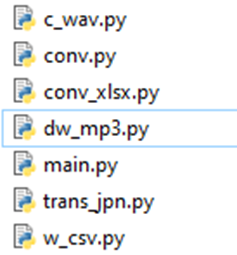
コード全文
main.py
import dw_mp3 import conv import c_wav import w_csv import conv_xlsx import trans_jpn name_mp3='test_voice.mp3' name_wav="test.wav" name_csv="test.csv" name_xlsx="test.xlsx" name_out_xlsx="test_out.xlsx" url = input('Enter URL:') dw_mp3.dw_mp3(url,name_mp3) conv.conv(name_mp3,name_wav) c_wav.c_wav(name_wav) w_csv.w_csv(name_csv,name_xlsx) conv_xlsx.conv_xlsx(name_csv,name_xlsx) trans_jpn.trans_jpn(name_xlsx,name_out_xlsx) print("全ての処理が完了しました")
dw_mp3.py
import youtube_dl import os from pathlib import Path def dw_mp3(url,name_mp3): # 初期設定 ydl = youtube_dl.YoutubeDL({'outtmpl': '%(id)s%(ext)s', 'postprocessors': [{ 'key': 'FFmpegExtractAudio', 'preferredcodec': 'mp3', 'preferredquality': '192', }] }) # 動画情報をダウンロードする with ydl: result = ydl.extract_info( url, download=True ) filename_before1 = url[32:] + 'mp4' + '.mp3' # ファイル名にwebmが付くのでその対応 filename_after1 = name_mp3 current_dir = Path(os.getcwd()) os.rename(current_dir/filename_before1, current_dir/filename_after1) print('音声ファイルのダウンロードが完了しました')
conv.py
import pydub def conv(name_mp3,name_wav): base_file=pydub.AudioSegment.from_mp3(name_mp3) base_file.export(name_wav,format="wav")
c_wav.py
import wave import struct import math import os from scipy import fromstring, int16 def c_wav(name_wav): #A列~ZZ列までの場合 def toAlpha2(num): i = int((num-1)/26) j = int(num-(i*26)) Alpha = '' for z in i,j: if z != 0: Alpha += chr(z+64) return Alpha #読み込むファイル名 f_name = name_wav #切り取り時間[sec] cut_time = 20 # 保存するフォルダの作成 file = os.path.exists("output") print(file) if file == False: os.mkdir("output") def wav_cut(name_wav,time): # ファイルを読み出し wavf = name_wav wr = wave.open(wavf, 'r') # waveファイルが持つ性質を取得 ch = wr.getnchannels() width = wr.getsampwidth() fr = wr.getframerate() fn = wr.getnframes() total_time = 1.0 * fn / fr integer = math.floor(total_time) # 小数点以下切り捨て t = int(time) # 秒数[sec] frames = int(ch * fr * t) num_cut = int(integer//t) # 確認用 print("Channel: ", ch) print("Sample width: ", width) print("Frame Rate: ", fr) print("Frame num: ", fn) print("Params: ", wr.getparams()) print("Total time: ", total_time) print("Total time(integer)",integer) print("Time: ", t) print("Frames: ", frames) print("Number of cut: ",num_cut) # waveの実データを取得し、数値化 data = wr.readframes(wr.getnframes()) wr.close() X = fromstring(data, dtype=int16) print(X) for i in range(num_cut): print(i) # 出力データを生成 outf = 'output/' + str(toAlpha2(i+1)) + '.wav' start_cut = i*frames end_cut = i*frames + frames print(start_cut) print(end_cut) Y = X[start_cut:end_cut] outd = struct.pack("h" * len(Y), *Y) # 書き出し ww = wave.open(outf, 'w') ww.setnchannels(ch) ww.setsampwidth(width) ww.setframerate(fr) ww.writeframes(outd) ww.close() wav_cut(f_name,cut_time)
w_csv.py
import csv import os import speech_recognition as sr import csv def w_csv(name_csv): count=0 with open(name_csv,'w') as f: writer = csv.writer(f) for fd_path, sb_folder, sb_file in os.walk('./output/'): for fil in sb_file: count= count+1 file_name=fd_path +fil r = sr.Recognizer() with sr.AudioFile(file_name) as source: audio = r.record(source) text = r.recognize_google(audio, language='ja-JP') # 英語の場合はen-US print(count,fil," 文字起こし完了") with open(name_csv, 'a', newline='',encoding="shift_jis") as csvfile: write = csv.writer(csvfile, delimiter=' ') write.writerow()
conv_xlsx.py
import openpyxl from openpyxl.styles.fonts import Font import pandas as pd def conv_xlsx(name_csv,name_xlsx): path = name_csv out = name_xlsx data =pd.read_csv(path) data.to_excel(out,index=False) wb = openpyxl.load_workbook(out) ws = wb['Sheet1'] font = Font(bold=False,name="Meiryo UI") for row in ws.iter_rows(): for cell in row: ws[cell.coordinate].font = font wb.save(out)
trans_jpn.py
from googletrans import Translator import openpyxl def trans_jpn(name_xlsx,name_out_xlsx): tr = Translator(service_urls=['translate.googleapis.com']) path = name_xlsx out = name_out_xlsx wb=openpyxl.load_workbook(path) sheet = wb.active max_row1=sheet.max_row max_row2=max_row1+1 for i in range(1,max_row2): print(i) trans = tr.translate(sheet.cell(i,1).value, dest="ja").text sheet.cell(i+1+max_row2,1).value = trans wb.save(out)
出力結果
上記のプログラムを実行した結果は↓です。
you might be wondering who this is so his name is Christine liwag I’m actually going to come back to her a bit later on in today’s video Butthole type everything will make sense in a bit okay let’s move on and again sorry for these shocking intro okay so Virgin Galactic stock is dropping we’re at about $27 today without |
15% almost 15% in today’s trading day alone and yesterday’s trading day we were down about 11% solenoid down about 25% from the highs on Monday of about $35 that why the significant drop in stock price with broken the support now and we’re going down below the support level |
and the next one really is about $25 out say about 23 to $25 23 to $25 so again why the sudden drop in the stock price check this out article some Morgan Stanley downgrades Virgin Galactic to underway and save data |
suction full 20% CNBC Market inside the Motley Fool that was saying it but check this out so Christine Levi downgraded the share of Virgin Galactic from equal-weight to underweight with a $25 price Target |
the game back to the first picture I showed you this is Christine liwag so I was just wondering how much are credentials does she have so how much should we trust her analytics so this is tipranks with it analyzed different analyst and you can see how this is Christine Levi from Morgan Stanley Wall Street |
list she has less than one star in terms of how popular she is as an analyst she’s actually ranked 6521 Oz 7617 LS that’s very low success rate is 52% that’s almost like flipping a coin if you want to trust |
she says average return is – 3.2% you better off putting your money in a bank and taking the 0.1% interest that they give you so yeah basically I’m saying is that we shouldn’t trust people like this but they went for Morgan Stanley serve for some reason the scene as quick or expose |
check this out from Reddit this was a Reddit post that came out just a few hours ago same what’s with the sudden drop and it obviously we know about the market manipulation going on now with Morgan Stanley but I couldn’t agree more with MMA said so this is an hour ago and ridiculous that they can do this |
they can shoot it and buy back cheaper making money in every direction it’s good to be the big guys so I completely agree with that what’s really happening is they basically manipulating Market in order to make money so what they saying now is that the stock price is too high and so they trying to show the stop meaning that they want to buy a |
price and hopefully profit on the way down by selling it when the stock is low and then pocketing the difference but how did they make the stock price go down what they do is they put out articles like endless reports from a from very bad and list as we can see what people listen the stock price goes down and they profit from that |
and they know any profit from the stock price going down what happens when the stock price goes down as they buy the stock and then ride the wave up with his well this is complete Market manipulation is someone like myself and yourself try to do this that would we put in jail for this but it’s okay for the big boys to do that for some reason I think it’s |
play unreasonable by a Hawkins week we can I demurred about it all week and see the positive in it the way I see the positive is that we’re going to continue riding the wave up with but when we do see pullbacks like this the way I see it is this a great buying opportunity because in the long run as long as Virgin Galactic continues to execute as they are |
executing them we’re likely to see the stock price be much higher than current levels today and as long as it’s higher than current levels today in the future then we will profit from it and that’s passed me how I see it I see it as. That’s how I look at it in a positive light is basically giving an opportunity for people |
haven’t been able to get into the stock at in order to actually buy at a decent price so positive in terms of how we see it I think it’s complete BS the fact that they they’ve use someone like Christine Lee Wags to analyze his company and come up with |
これが誰なのか疑問に思われるかもしれませんが、彼の名前はChristineliwagです。今日のビデオで少し後で彼女に戻ってきます。バットホールタイプは少し意味があります。先に進みましょう。これらの衝撃的なイントロをもう一度申し訳ありません。さて、ヴァージンギャラクティックの株価は下落しています。今日は約27ドルです。 |
今日の取引日だけで15%、ほぼ15%、昨日の取引日では、月曜日の最高値である約35ドルから約11%ソレノイドが約25%低下しました。そのため、株価が大幅に下落し、現在のサポートが壊れています。サポートレベルを下回っています |
次は実際には約25ドルです。たとえば23ドルから25ドル23ドルから25ドルです。なぜ株価が急落したのか、この記事をチェックしてください。モルガンスタンレーがヴァージンギャラクティックをダウングレードしてデータを保存しています。 |
それを言っていたMotleyFool内の完全な20%CNBCマーケットを吸引しますが、これをチェックして、ChristineLeviがVirginGalacticのシェアを25ドルの価格で同等のウェイトからアンダーウェイトにダウングレードしました目標 |
最初の写真に戻って、これがChristine liwagであることを示したので、彼女はどのくらいの資格を持っているので、彼女の分析をどれだけ信頼する必要があるのか疑問に思っていました。モルガンスタンレーウォールストリートのクリスティンレヴィ |
リスト彼女はアナリストとしての人気の点で星が1つ未満です。彼女は実際に6521Oz 7617 LSにランク付けされています。成功率は52%と非常に低く、信頼したい場合はコインを投げるようなものです。 |
彼女は、平均収益率は-3.2%だと言っています。銀行にお金を入れて、0.1%の利子をとったほうがいいので、基本的には、このような人を信用すべきではないと言っていますが、彼らはモルガンスタンレーに行きました。何らかの理由でシーンをすばやく公開する |
Redditからこれをチェックしてくださいこれはほんの数時間前に出てきたRedditの投稿で、突然の落ち込みと同じで、モルガンスタンレーで現在行われている相場操縦については明らかに知っていますが、MMAがそう言ったことにこれ以上同意できませんでしたこれは1時間前で、彼らがこれを行うことができるのはばかげています |
彼らはそれを撃ち、あらゆる方向でより安くお金を稼ぐことができるので、大物になるのは良いことです。だから私は完全に同意します。彼らは基本的に市場を操作してお金を稼ぐので、彼らが今言っているのは株価は高すぎるので、彼らはストップを見せようとしているので、彼らは購入したいという意味です |
価格とうまくいけば、在庫が少ないときにそれを売って差額をポケットに入れることで、途中で利益を上げますが、どうやって株価を下げたのですか?彼らは非常に悪いからの無限のレポートのような記事を出し、株価が下がるのを聞いている人がいるのがわかります。 |
そして彼らは株価が下がることによる利益を知っています。彼らが株を購入し、それから彼の井戸で波に乗るときに株価が下がるとどうなるかこれは完全です市場操作は私のような誰かであり、あなた自身がこれをやろうとします私たちはこれのために刑務所に入れましたが、大きな男の子が何らかの理由でそれをするのは大丈夫だと思います |
ホーキンスの週までに不合理にプレーする私たちはそれについて一週間中非難し、ポジティブを見ることができますポジティブを見る方法は私たちが波に乗り続けることですが、私たちがこのように引き戻しを見るとき私はヴァージンギャラクティックがそのまま実行し続ける限り、長期的にはこれは素晴らしい購入の機会であることがわかります |
それらを実行すると、株価は現在の水準よりもはるかに高くなる可能性があり、将来的には現在の水準よりも高い限り、それから利益を得ることができます。それが私が前向きに見ている方法であり、基本的に人々に機会を与えています |
実際にまともな価格で購入するために在庫に入ることができなかったので、私たちの見方の点で非常にポジティブです。彼らがクリスティン・リー・ワグスのような誰かを使って彼を分析したという事実は完全なBSだと思います会社と思い付く |
意図通り、英語を日本語に翻訳して出力することができました!
以上です!!
コメント