こんにちは。
今日は、PythonのFraskで簡易的なif文を使用したクイズアプリの作り方を書きます。
誰かの役に立ったら幸いです。
概要
ざっと構成を書くと以下のような感じ。
①GET/POSTメソッドを使用して2ページ用意する
(問題出題と回答後でhtmlを2つ用意しておく)
②if文を利用し、選択肢によって回答後に表示する文字を変更する
コードは一番下に載せておくので、要点から先に説明していく。
完成した姿
先に完成した姿を載せておく。
問題出題
↓のようにクイズを出題し、チェックを入れて回答できるようにしている。

正解した場合
正解(桶狭間の戦い)を選択してAswerを押した場合、正解!!を表示する
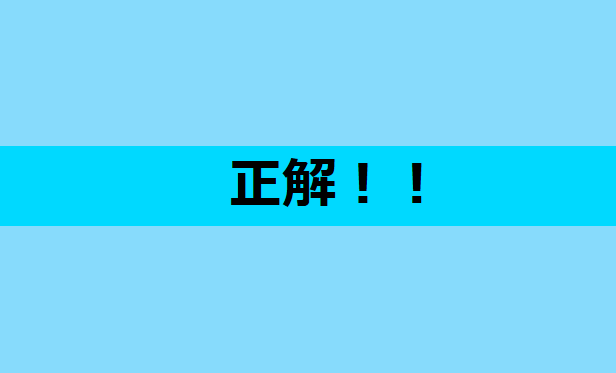
不正解の場合
不正解(関ケ原 or 長篠の戦い)を選んでAswerを押した場合、↓のように不正解と表示する
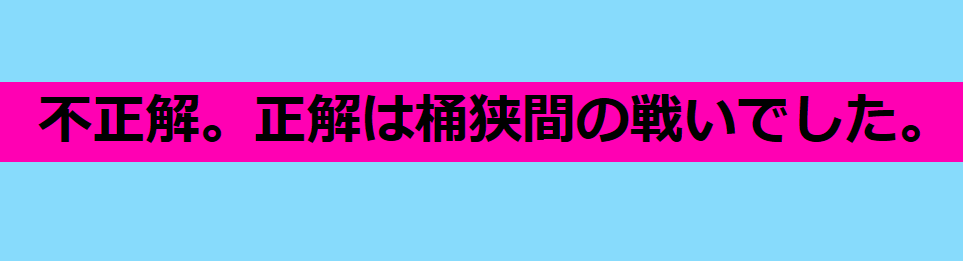
①GET/POSTメソッドを使用して2ページ用意する
以下のようにget/postを使用して、問題出題と回答後でhtmlを2ページ用意する
@app.route(‘/’, methods=[‘GET’])
def index():
return render_template(‘index.html‘, \
title=”Welcome to Rope_Blog”,\
message=”織田信長が今川義元を打ち取った戦いの名は?”,\
)
@app.route(‘/’, methods=[‘POST’])
def form():
rd = request.form.get(‘radio’)
return render_template(‘index2_post.html‘,\
)
return render_template()
render_template関数の()内の引数には、利用するファイル名を指定する。
ファイルは[templates]フォルダの名から検索して、読み込みんだファイルをreturnする。
つまり、GETとPOST内でretrun(表示)するhtmlを分けることができる。
②if文を利用し、選択肢によって回答後に表示する文字を変更する
回答前(最初)のHTML
選択肢によってvalueを定義する。
関ケ原の戦いを選んだ場合は、value=a
桶狭間の戦いを選んだ場合は、value=b
長篠の戦いを選んだ場合は、value=c
のように定義した。
↓のコードは回答前のhtmlのコードの抜粋。
HTML(ファイル名:index.html)の抜粋
<form method=”post” action=”/”>
<div>
<input type=”radio” name=”radio” id=”r1″ value=a style=”width:25px;height:25px;”>
<label for =”r1″ style=”font-size:30pt;”>
関ケ原の戦い</label>
</div>
<div>
<input type=”radio” name=”radio” id=”r2″ value=b style=”width:25px;height:25px;”>
<label for =”r2″ style=”font-size:30pt”>
桶狭間の戦い</label>
</div>
<div>
<input type=”radio” name=”radio” id=”r3″ value=c style=”width:25px;height:25px;”>
<label for =”r3″ style=”font-size:30pt”>
長篠の戦い</label>
</div>
回答後のHTML
選択肢(value)によって、表示する文字列を変更する。
if文を利用して、変数の値に応じて表示する文字列を変更する。
変数がbの時は、正解!!
変数がb以外の時は、不正解。正解は桶狭間の戦いでした。
と表示できるようにした
HTML(ファイル名:index_post.html)の抜粋。
{% if flg == ‘a’ %}
<div class=”flg1″>
<p style=”font-size:60pt;background-color:rgb(255, 0, 179); font-weight:bold; “>不正解。正解は桶狭間の戦いでした。</p>
</div>
{% elif flg == ‘b’ %}
<div class=”flg2″>
<p style=”font-size:60pt;background-color:rgb(0, 217, 255); font-weight:bold; “>正解!!</p>
</div>
{% else %}
<div class= “flg3″>
<p style=”font-size:60pt;background-color:rgb(255, 0, 179); font-weight:bold; “>不正解。正解は桶狭間の戦いでした。</p>
</div>
Python(Frask)のコード
if文で使用する変数の引き渡しを行う。
flg=rd を定義して、GETとPOST間の引き渡しを行う。
.pyファイル(if文に関係する箇所を赤字で記載する)
@app.route(‘/’, methods=[‘POST’])def form():
flg= ‘rd’
rd = request.form.get(‘radio’)
return render_template(‘index2_post.html’,\
flg=rd)
ハマったこと
Valueの適宜を数字(1とか2)にすると上手くいかなかった。
英数字にすると上手くいった。
全コード
一応、全文のコードも載せておく
.py(python_frask)ファイル
from flask import Flask, render_template, request
app = Flask(name)
@app.route(‘/’, methods=[‘GET’])
def index():
return render_template(‘index.html’, \
title=”Welcome to Rope_Blog”,\
message=”織田信長が今川義元を打ち取った戦いの名は?”,\
)
@app.route(‘/’, methods=[‘POST’])
def form():
flg= ‘rd’
rd = request.form.get(‘radio’)
return render_template(‘index2_post.html’,\
flg=rd)
if name == ‘main‘:
app.debug=True
app.run(),
CSS
html,body {height:100%;}
div.test{
display:table;
width:100%;
height:100%;
background-color:rgb(135, 219, 252);
text-align:center;
}
div.test .target{
display:table-cell;
vertical-align:middle;
}
h1 {
color: rgb(41, 61, 245);
font-size: 42pt;
margin:0px;
}
div.subtitle p{
font-size:36pt;
font-weight:bold;
background-color:rgb(145, 255, 0)
}
回答前のhtml
<!doctype html>
<html lang=”ja”>
<head>
<title>Index </title>
<meta charse=”utf-8″/>
<link rel=”stylesheet”
href=”{{url_for(‘static’,filename=’style.css’)}}”>
</head>
</html>
<body>
<div class=”test”>
<span class=”target”>
<h1>{{title}}</h1>
<div class=”subtitle”>
<p>This is quiz</p>
</div>
<p style=” font-size:36pt”>
{{message}}</p>
<form method=”post” action=”/”>
<div>
<input type=”radio” name=”radio” id=”r1″ value=a style=”width:25px;height:25px;”>
<label for =”r1″ style=”font-size:30pt;”>
関ケ原の戦い</label>
</div>
<div>
<input type=”radio” name=”radio” id=”r2″ value=b style=”width:25px;height:25px;”>
<label for =”r2″ style=”font-size:30pt”>
桶狭間の戦い</label>
</div>
<div>
<input type=”radio” name=”radio” id=”r3″ value=c style=”width:25px;height:25px;”>
<label for =”r3″ style=”font-size:30pt”>
長篠の戦い</label>
</div>
<div class=”submit”>
<input type=”submit”value =”Answer” style=”width:200px; height:50px; font-size:20pt; margin: 50px 0px; background-color: rgb(86, 204, 240); font-weight:bold”>
</div>
</form>
</span>
</dvi>
</body>
</hmtl>
回答後のHTML
!doctype html>
<html lang=”ja”>
<head>
<title>Index </title>
<meta charse=”utf-8″/>
<link rel=”stylesheet”
href=”{{url_for(‘static’,filename=’style.css’)}}”>
</head>
</html>
<body>
<div class=”test”>
<span class=”target”>
<h1>{{title}}</h1>
{% if flg == ‘a’ %}
<div class=”flg1″>
<p style=”font-size:60pt;background-color:rgb(255, 0, 179); font-weight:bold; “>不正解。正解は桶狭間の戦いでした。</p>
</div>
{% elif flg == ‘b’ %}
<div class=”flg2″>
<p style=”font-size:60pt;background-color:rgb(0, 217, 255); font-weight:bold; “>正解!!</p>
</div>
{% else %}
<div class= “flg3”>
<p style=”font-size:60pt;background-color:rgb(255, 0, 179); font-weight:bold; “>不正解。正解は桶狭間の戦いでした。</p>
</div>
{% endif %}
<p style=” font-size:36pt”>
{{message}}</p>
</span>
</dvi>
</body>
</hmtl>
コメント